Python3 connection to SonicOS API using only urllib
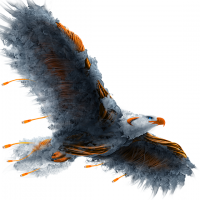
I only have urllib available, not requests or urllib2/3. I am not able to authenticate - HTTP Error 401: Unauthorized error. Is there reference code for authentication using urllib? Here's my code:
import urllib
import urllib.request
import ssl
from collections import OrderedDict
_api_auth = ("xxxx", "xxxx")
_firewall_url = 'xxxx'
_context = ssl._create_unverified_context()
def auth():
_url = _firewall_url + 'api/sonicos/auth'
_auth_headers = OrderedDict([
('Accept', 'application/json'),
('Content-Type', 'application/json'),
('Accept-Encoding', 'application/json'),
('charset', 'UTF-8')])
_auth_request = urllib.request.Request(_url, headers=_auth_headers)
try:
password_mgr = urllib.request.HTTPPasswordMgrWithDefaultRealm()
password_mgr.add_password(None, _url, _api_auth[0], _api_auth[1])
handler = urllib.request.HTTPBasicAuthHandler(password_mgr)
opener = urllib.request.build_opener(handler) urllib.request.install_opener(opener)
result = urllib.request.urlopen(_auth_request, context=_context)
except urllib.error.URLError as e:
print('Error:', e)
else:
print("Everything ok")
print(result.status)
def main():
auth()
if __name__ == "__main__"
main()
Best Answer
-
Jaime SonicWall Employee
Please give this a try.
import urllib.request
from base64 import b64encode
from collections import OrderedDict
import ssl
# Replace these with your SonicOS API endpoint and credentials
sonicos_api_url = "https://xxx.xxx.xxx.xxx/api/sonicos/"
username = "admin-user"
password = "admin-password"
# Encode the username and password for Basic Authentication
credentials = f"{username}:{password}"
encoded_credentials = b64encode(credentials.encode("utf-8")).decode("utf-8")
# Set up the request with the necessary headers
headers = {
"Authorization": f"Basic {encoded_credentials}",
"Content-Type": "application/json",
}
# Create a request object
context = ssl._create_unverified_context()
request = urllib.request.Request(sonicos_api_url + "auth", headers=headers, method='POST')
# Send the request and get the response
try:
with urllib.request.urlopen(request, context=context) as response:
response_data = response.read().decode('utf-8')
print("Successfully authenticated!")
print("Response data:", response_data)
except urllib.error.HTTPError as e:
print(e)
print(f"Failed to authenticate. Status code: {e.code}")
print("Response:", e.read().decode('utf-8'))
except urllib.error.URLError as e:
print("Failed to connect to the server. Error:", e.reason)
request = urllib.request.Request(sonicos_api_url + "version")
try:
with urllib.request.urlopen(request, context=context) as response:
response_data = response.read().decode('utf-8')
print("Response data:", response_data)
except urllib.error.HTTPError as e:
print(e)
print("Response:", e.read().decode('utf-8'))
except urllib.error.URLError as e:
print("Failed to connect to the server. Error:", e.reason)As an aside, there's probably methods of getting pip installed. Take a look at
0
Answers
Please verify that Basic Authentication is enabled in your SonicOS API settings. I believe Basic HTTP authentication is disabled by default.
Also, why not 'pip install requests'?
Yes, I have enabled Basic Authentication in API settings. I am working with a very limited functionality linux OS which does not even have pip installed.
Thank you that works!
Is there an example of python code using HTTP digest authentication with urllib?
I've only attempted the more advanced auth settings with the requests library.